Machine Learning with Laravel and AWS Personalize Part 3
In Part 1, we set up the Laravel project and the initial models and relationships.
In Part 2, we configured AWS Personalize and set up the data groups so that Personalize is able to process interactions and start building recommendations for our users.
AWS Personlize Solutions
Solutions are pre configured recipes that the AWS team have built based on their experiences of Amazon Retail.
Some of the pre-configured recipes are:
-
SIMS Computes items similar to a given item based on co-occurrence of items in the user-item interactions dataset.
-
SIMS (NEW) Predicts items similar to a given item based on co-occurrence of items in the user-item interactions dataset and item metadata in the item dataset.
-
Personalized Ranking Reranks an input list of items for a given user. Trains on user-item interactions dataset, item metadata and user metadata.
-
User Personalization Predicts items a user will interact with and performs exploration on cold items. Based on Hierarchical Recurrent Neural Networks which model the temporal order of user-item interactions.
-
Popularity Count Calculates popularity of items based on total number of events for each item in the user-item interactions dataset.
Create a Personalized Solution
We'll start by using Personalized Personalization Recipe to test out our datasets.
For the Solution Name
user-personalization
Solution Type
Item Recommendation
Recipe
aws-user-personalization
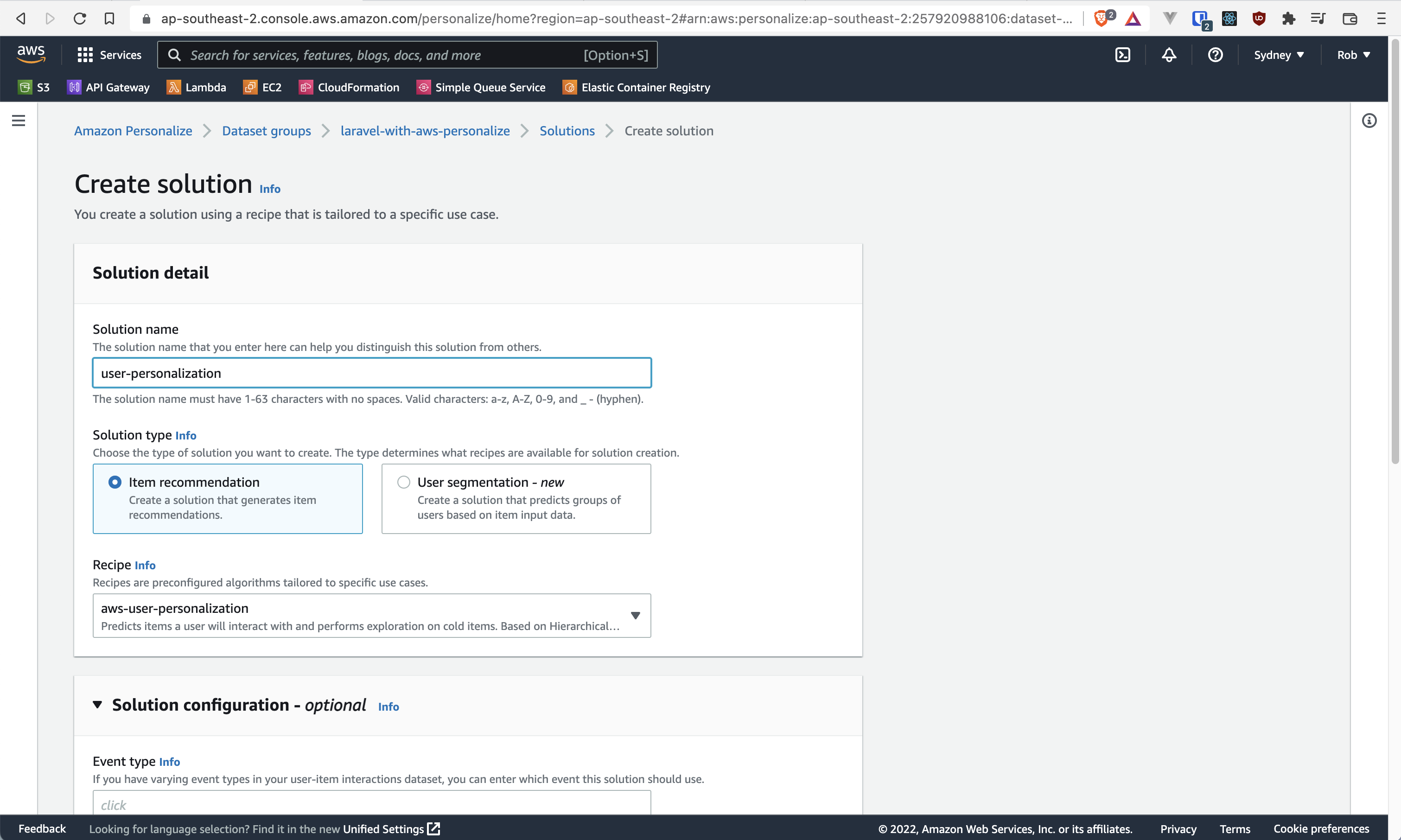
You can skip the optional settings, I suggest reading up on what they do though.
Finish up by clicking on the Create and train solution button. Amazon is now running it's machine learning algorithms across your data.
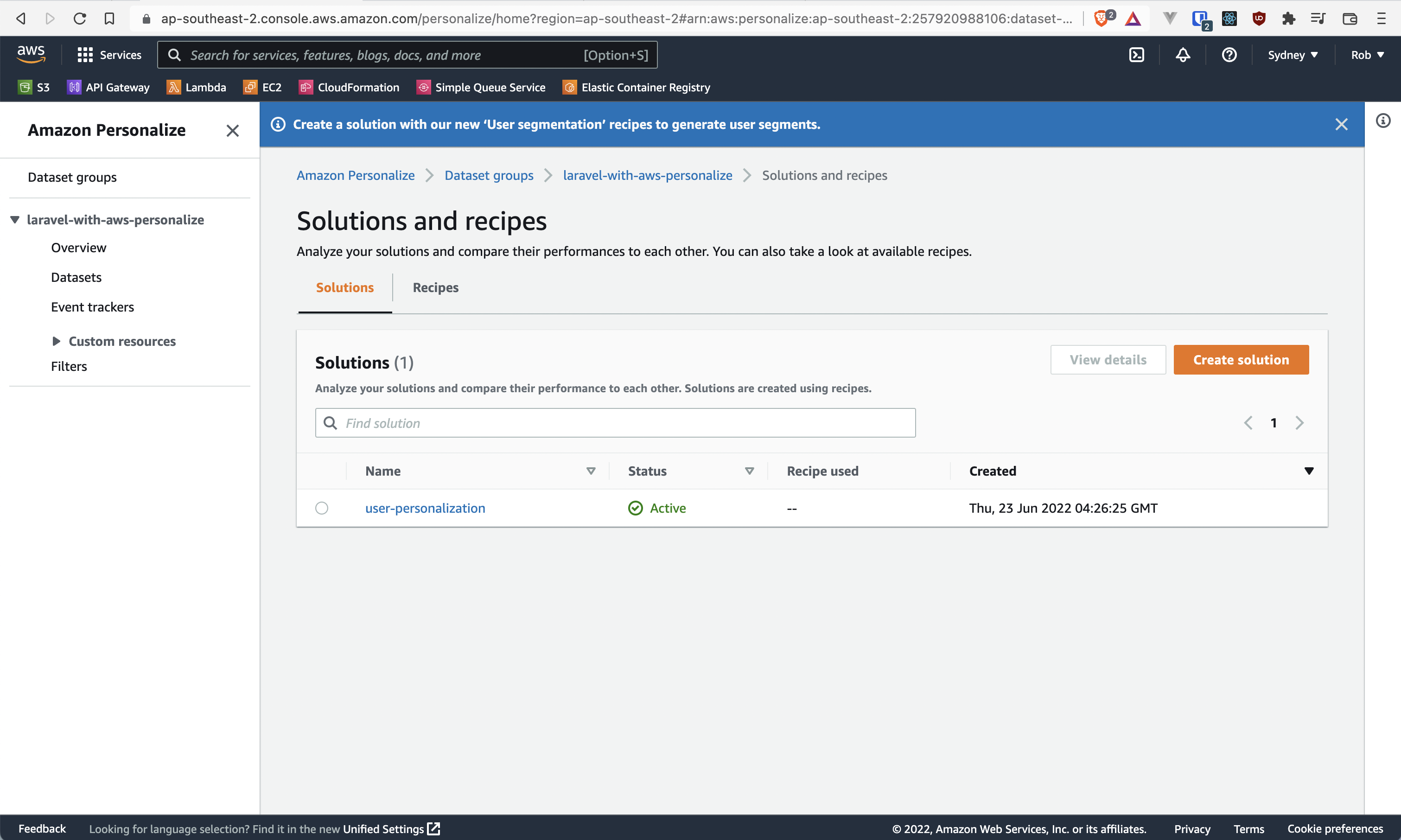
Create a Personalized Campaign
When the previous step has complete, and AWS has finished processing the data, you will be able to create a Campaign around the solution.
Enter the following details
Campaign Name: personalization-campaign
For the solution, select the solution from the previous step.
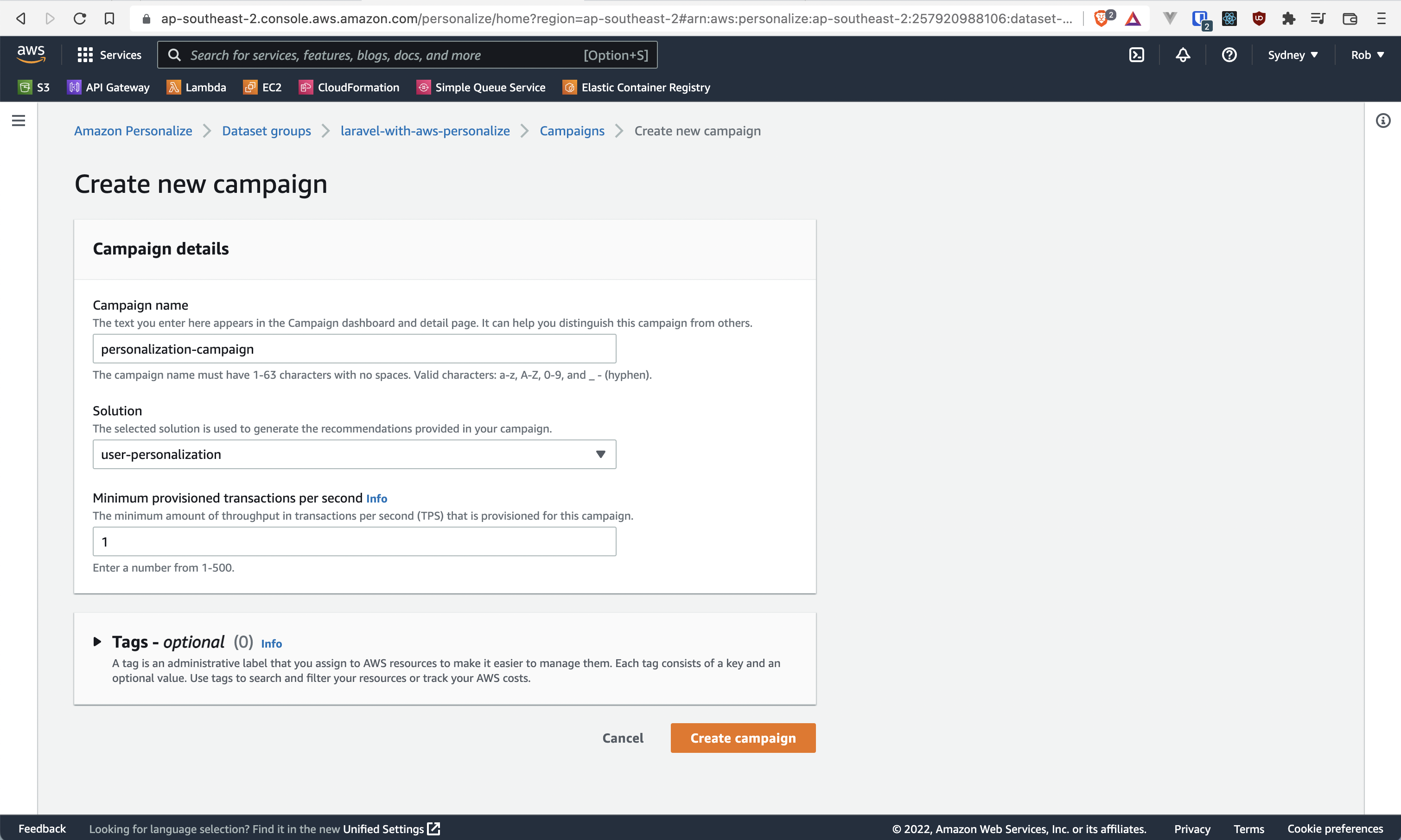
When you're finished press Create Campaign
On the next screen you will see the Campaign creation in progress.
Make sure to copy the Campaign ARN, as we'll need this to connect Laravel to the campaign.
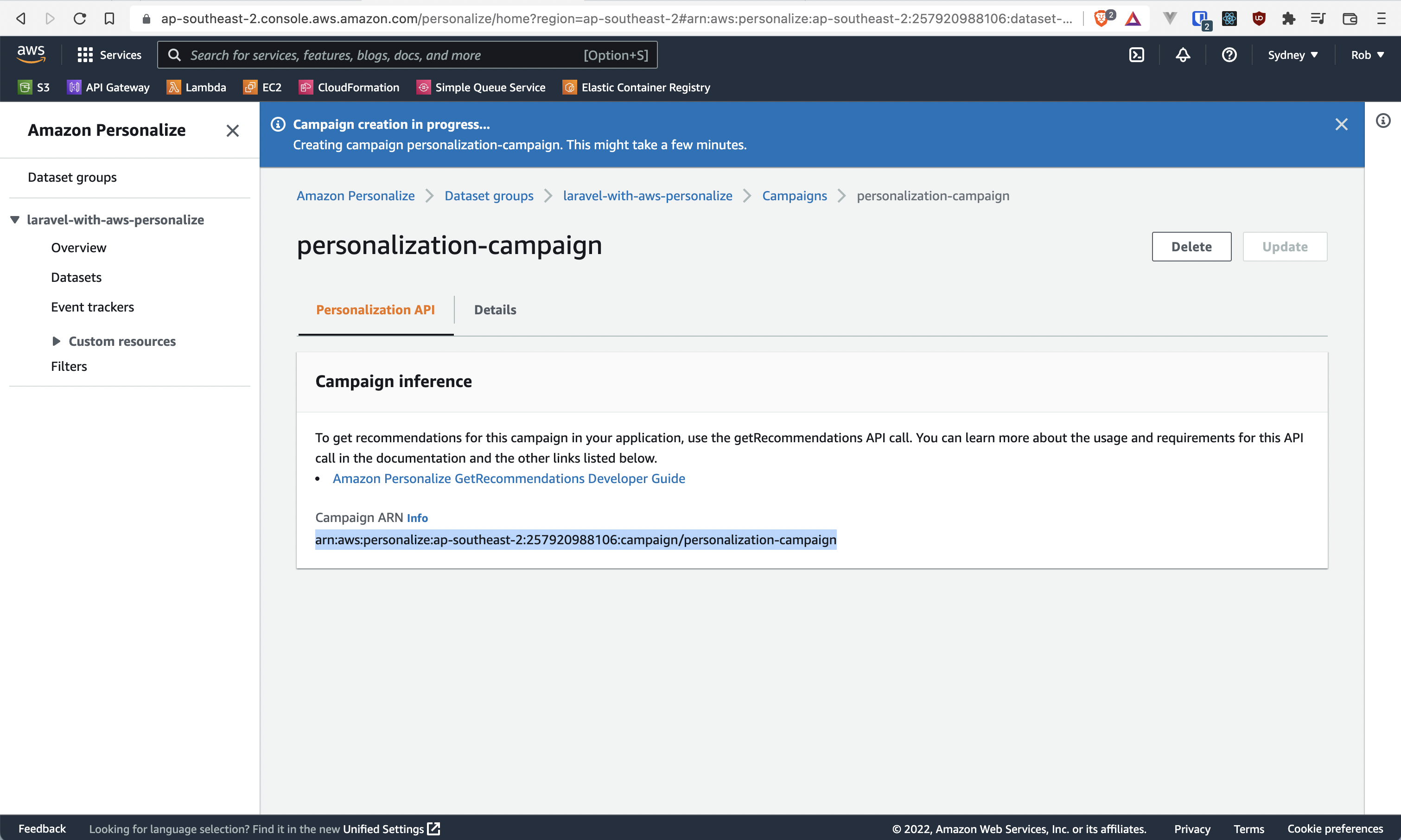
This will take about 15 minutes to create.
Configuring Laravel to Interact with AWS Personalize
Next up, we'll need to install the Amazon PHP SDK.
composer require aws/aws-sdk-php
In our services config, we'll add a section for AWS Personalize.
// config/services.php
'aws_personalize' => [
'access_key_id' => env('AWS_ACCESS_KEY_ID'),
'access_key' => env('AWS_SECRET_ACCESS_KEY'),
'default_region' => env('AWS_DEFAULT_REGION'),
'campaign_arn' => env('AWS_PERSONALIZE_CAMPAIGN_ARN')
],
Make sure you populate your .env
with the following variables
AWS_ACCESS_KEY_ID
AWS_SECRET_ACCESS_KEY
AWS_DEFAULT_REGION
AWS_PERSONALIZE_CAMPAIGN_ARN
and the value of the campaign in the previous step.
We'll create a Service Provider to interact with the Recommendations.
// app/Providers/AppServiceProvider.php
/**
* Register any application services.
*
* @return void
*/
public function register()
{
$this->app->bind(PersonalizeRuntimeClient::class, function ($app) {
return new PersonalizeRuntimeClient([
'version' => 'latest',
'region' => config('services.aws_personalize.default_region'),
'credentials' => [
'key' => config('services.aws_personalize.access_key_id'),
'secret' => config('services.aws_personalize.access_key'),
],
]);
});
}
Now we'll create a controller to fetch the recommendations from AWS Personalize.
<?php
namespace App\Http\Controllers;
use App\Models\Movie;
use Aws\PersonalizeRuntime\PersonalizeRuntimeClient;
use Illuminate\Http\Request;
class RecommendationController extends Controller
{
private PersonalizeRuntimeClient $service;
public function __construct(PersonalizeRuntimeClient $personalizeService)
{
$this->service = $personalizeService;
}
public function __invoke(Request $request)
{
$recommendations = $this->service->getRecommendations([
'campaignArn' => config('services.aws_personalize.campaign_arn'),
'userId' => (string) $request->id,
'numResults' => 500
]);
return response()->json(
Movie::whereIn('id', data_get($recommendations->toArray(), 'itemList.*.itemId'))->get()
);
}
}
In Insomnia or Postman, if you fire a http request at your new endpoint /user/{id}/recommendations
, you should be able to see a list of recommended movies for the user!
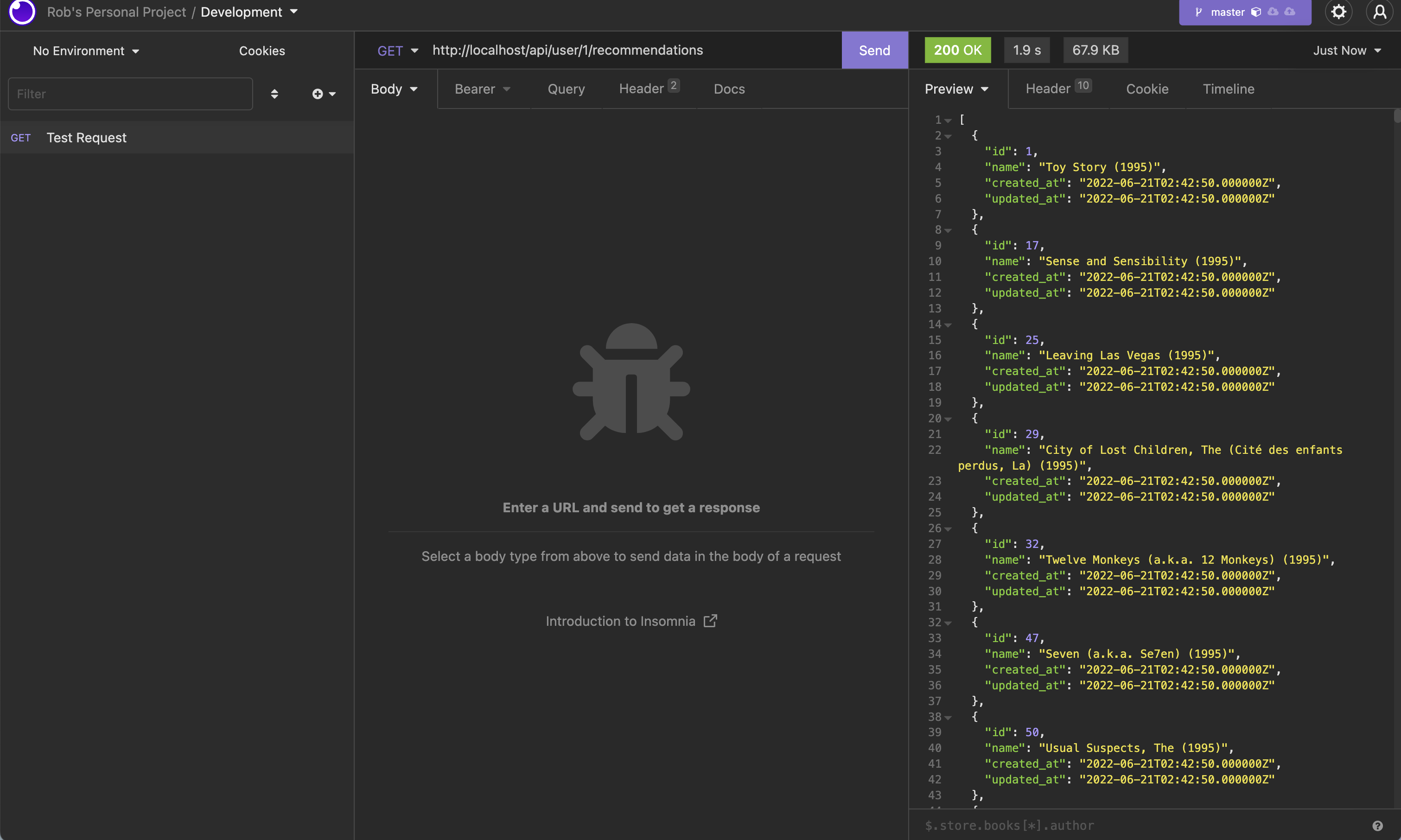
This is just scratching the surface of what's possible in AWS, they do provide a few more recipes and customization options we don't have time to explore right now.
If you enjoyed this tutorial follow me on LinkedIn or Twitter!